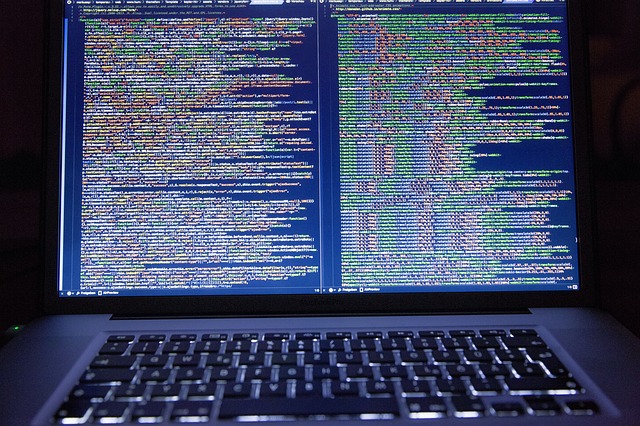
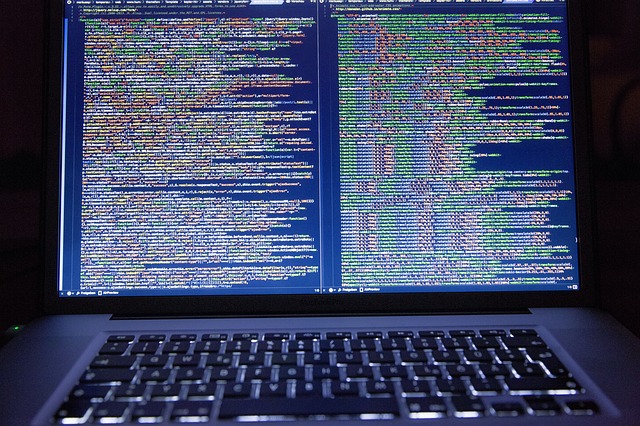
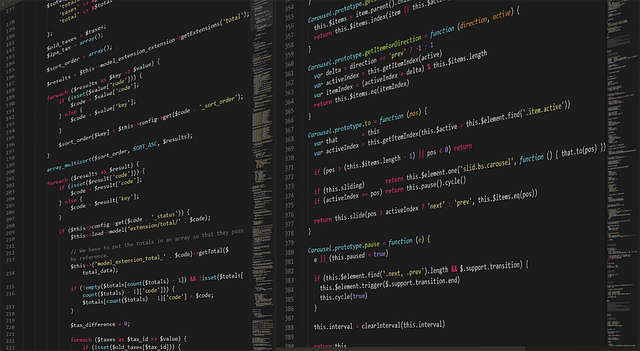
My solution to HackerRank Challenge “Queues: A Tale of Two Stacks”
This is my solution to challenge “Queues: A Tale of Two Stacks” on HackerRank. Click here to see the challenge. import java.io.*; import java.util.*; import java.text.*; import java.math.*; import java.util.regex.*; public class Solution { public static...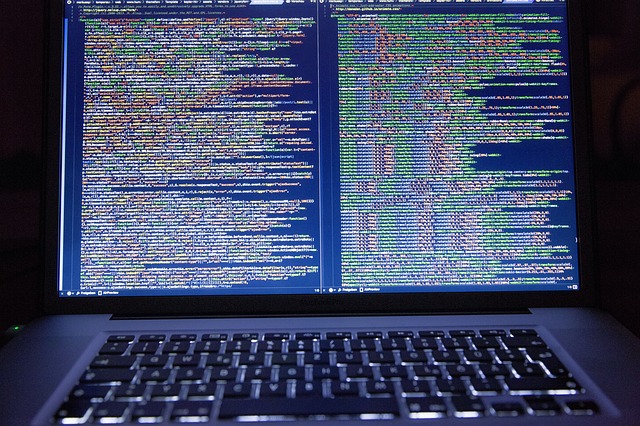
My solution to HackerRank Challenge “Stacks: Balanced Brackets”
This is my solution to challenge “Stacks: Balanced Brackets” on HackerRank. Click here to see the challenge. import java.io.*; import java.util.*; import java.text.*; import java.math.*; import java.util.regex.*; public class Solution { static HashMap<...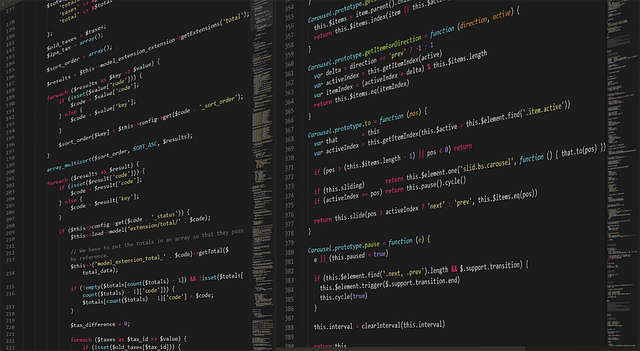
My solution to HackerRank Challenge “Hash Tables: Ransom Note”
This is my solution to challenge “Hash Tables: Ransom Note” on HackerRank. Click here to see the challenge. import java.io.*; import java.util.*; import java.text.*; import java.math.*; import java.util.regex.*; public class Solution { public static void...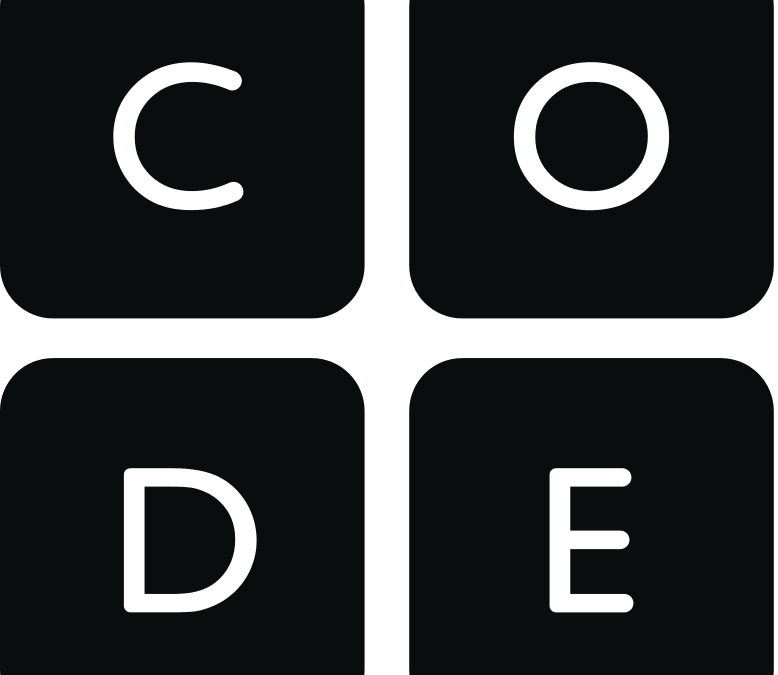
My solution to HackerRank Challenge “Strings: Making Anagrams”
This is my solution to challenge “Strings: Making Anagrams” on HackerRank. Click here to see the challenge. import java.io.*; import java.util.*; import java.text.*; import java.math.*; import java.util.regex.*; public class Solution { public static int...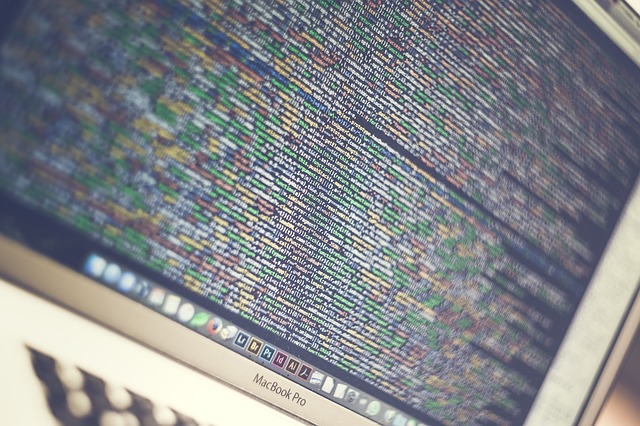
My solution to HackerRank Challenge “Trees: Is This a Binary Search Tree?”
This is my solution to challenge “Trees: Is This a Binary Search Tree?” on HackerRank. Click here to see the challenge. import java.io.*; /* Hidden stub code will pass a root argument to the function below. Complete the function to solve the challenge....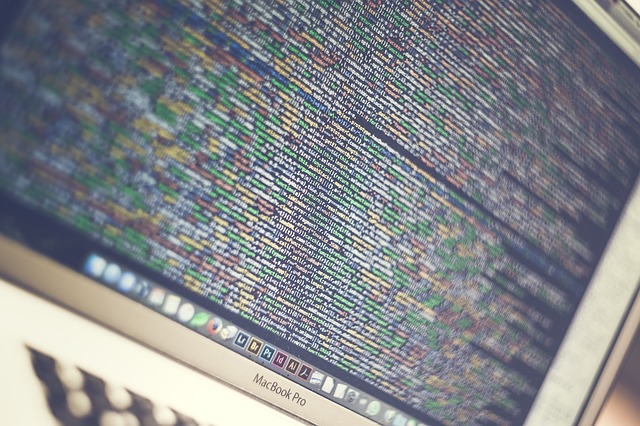
My solution to Arrays: Left Rotation Challenge on Hacker Rank
This is my solution to challenge about Arrays left rotation. Click here to see the challenge. import java.io.*; import java.io.*; import java.util.*; import java.text.*; import java.math.*; import java.util.regex.*; public class Solution { public static void...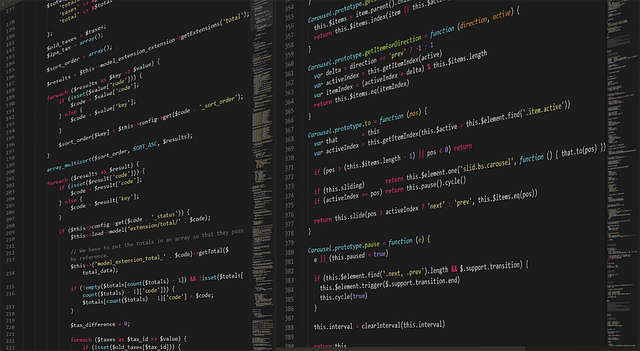
My solution to Tries: Contacts Challenge on Hacker Rank
This is my solution to challenge about the “Tries” data structure. Click here to see the challenge. import java.io.*; import java.util.*; import java.text.*; import java.math.*; import java.util.regex.*; class Node{ Node[] children = new...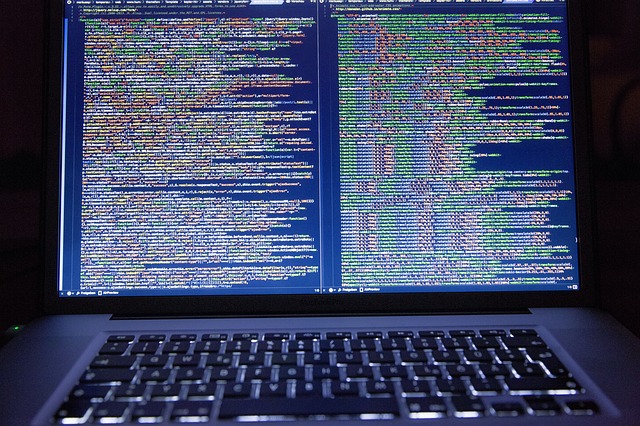